728x90
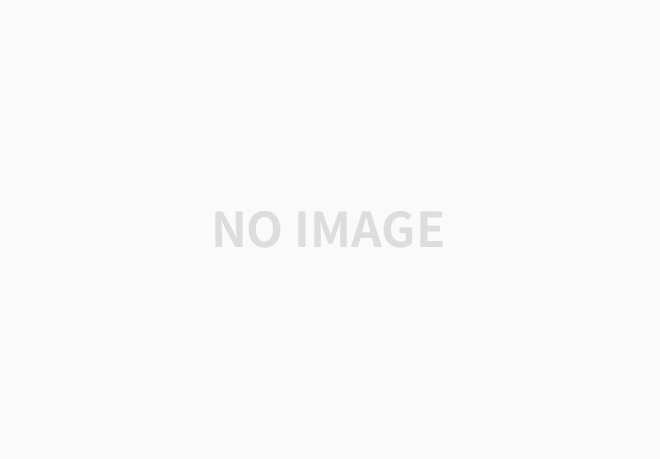
select country from customers; -- 모든 나라 나옴
distinct
중복없이 select를 해라
select country from customers; -- 모든 나라 나옴
select distinct country from customers; -- 나라 중복 삭제
select count(distinct country) from customers; -- 집게 함수
select count(*)
from (select distinct country from customers) as c; -- 괄호 안은 sub query
select * from customers
where country = 'mexico';
select * from customers
where customerid>80;
select*from products
where price = 18;
select * from products
where price between 50 and 60;
select * from customers
where city in ('paris','london');
select * from products
order by price asc; -- asc 올림차순, dsc 내림차순'
select * from customers
order by country asc, customername desc;
select * from customers
where customername like 'a%'; -- a로 시작하는 거 나열
select * from customers
where city like 'L_nd__'; -- 저 글자 포함된거 찾는거
select * from customers
where city like '%L%'; -- L 포함하는 경우만 선택
select * from customers
where customername like '%or%';
select * from customers
where customername like 'a__%';
select * from customers
where Country = 'spain';
select *
from customers
where country = 'Spain' and customername like 'G%';
select * from cusomters
where country = 'Spain' and customerName like 'G%' or cusomtername like 'R%';
select customername, contactname, address
from customers
where address is null;
SELECT *
from customers
limit 3;
select max(price) as "maxPrice"
from products;
select min(price) as SmallestPrice, categoryId
from products
group by categoryid
SELECT o.OrderID, o.OrderDate, c.CustomerName
FROM Orders o
INNER JOIN Customers c
ON o.CustomerID = c.CustomerID;
하나하나 파헤치자!
1. 서버 구축
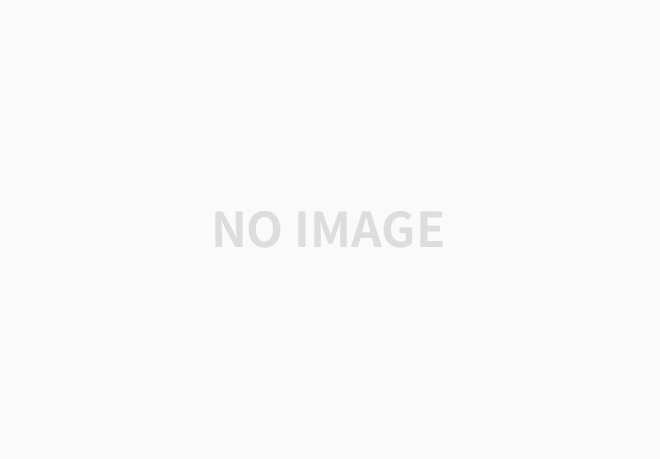
package com.shop.cafe.controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController // 특정 응답 controller를 갖지 않음
public class ProductController {
@GetMapping("getAllProducts") // 클라이언트가 get요청을 보낼 수 있도록 매필하는 역할
public String getAllProducts() {
return "ok";
}
}
🧐 @RestController는 왜 특정 응답 controller를 갖지 않을까?
→ 특정 HTML을 반환하지 않고 데이터를 직접 반환한다.
Controller의 역할은 클라이언트의 요청을 받아서 처리하는 역할
ProductController는 클라이언트가 특정 URL(getAllProducts)로 요청을 보낼때 이를 처리하고 응답을 돌려주는 역할을 한다.
getAllProducts 메서드의 역할은 위에 적혀있듯이 클라이언트가 URL로 GET요청을 보낼 수 있도록 정의된 메서드
요청받으면 "OK"라는 문자열을 응답하여 반환한다.
2. 데이터베이스 구축
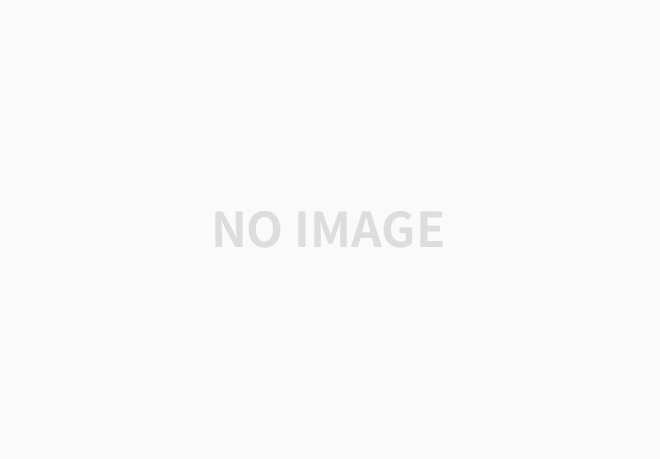
use ureca;
drop table if exists product;
create table product(
prodcode int auto_increment , -- auto_increment: 자동 숫자 증가, 1씩 증가
prodname varchar(50) unique not null, -- null 값 허용 x, unique: 중복 허용x
pimg varchar(50) ,
price int not null,
primary key (prodcode)
);
INSERT INTO `product` (`prodcode`,`prodname`,`price`,`pimg`) VALUES (1,'아이스 아메리카노',2700,'아이스아메리카노.png');
INSERT INTO `product` (`prodcode`,`prodname`,`price`,`pimg`) VALUES (2,'아이스 카페라떼',3500,'아이스카페라떼.png');
INSERT INTO `product` (`prodcode`,`prodname`,`price`,`pimg`) VALUES (3,'아이스 카푸치노',3800,'아이스카푸치노.png');
INSERT INTO `product` (`prodcode`,`prodname`,`price`,`pimg`) VALUES (4,'따듯한 아메리카노',2500,'핫아메리카노.png');
INSERT INTO `product` (`prodcode`,`prodname`,`price`,`pimg`) VALUES (5,'따듯한 카페라떼',3200,'핫카페라떼.png');
INSERT INTO `product` (`prodcode`,`prodname`,`price`,`pimg`) VALUES (6,'따듯한 카푸치노',3500,'핫카푸치노.png');
commit;
난 생크림 올라간 따듯한 카푸치노가 먹고 싶다.
3. 다시 서버 구축
package com.shop.cafe.dto;
public class Product {
private int prodcode, price; // 상품 코드와 가격 저장
private String prodname, pimg; // 상품 이름과 상품 이미지 저장
// 기본 생성자 만들기
// 왜 있어야 하냐? spring boot와 같은 프레임 워크들은 default 생성자를 갖고 일을 한다. 그래서 super키가 꼭 있어야한다.
public Product() {
super();
}
// 모든 정보를 받아서 객체를 만드는 생성자
public Product(int prodcode, int price, String prodname, String pimg) {
super();
this.prodcode = prodcode;
this.price = price;
this.prodname = prodname;
this.pimg = pimg;
}
// f/w는 외부에 있기에 public해야함
// 상품 코드 값을 가져오는 함수
public int getProdcode() {
return prodcode;
}
// 상품 코드 값을 설정하는 함수
public void setProdcode(int prodcode) {
this.prodcode = prodcode;
}
// 상품 가격 값을 가져오는 함수
public int getPrice() {
return price;
}
// 상품 가격 값을 설정하는 함수
public void setPrice(int price) {
this.price = price;
}
// 상품 이름 값을 가져오는 함수
public String getProdname() {
return prodname;
}
// 상품 이름 값을 설정하는 함수
public void setProdname(String prodname) {
this.prodname = prodname;
}
// 상품 이미지 값을 가져오는 함수
public String getPimg() {
return pimg;
}
// 상품 이미지 값을 설정하는 함수
public void setPimg(String pimg) {
this.pimg = pimg;
}
// toString 메서드가 있으면 무엇이 들어간지 확인할 수 있기에 좋다.
// 객체의 정보를 출력할 때 어떤 내용을 보여줄지 설정
@Override
public String toString() {
return "Product [prodcode=" + prodcode + ", price=" + price + ", prodname=" + prodname + ", pimg=" + pimg + "]";
}
}
package com.shop.cafe.dao; // 패키지 선언
import java.sql.*; // SQL 관련 클래스
import java.util.*; // Java의 컬렉션 클래스
import org.springframework.stereotype.Component;
import com.shop.cafe.dto.Product;// product 클래스를 가져와서 사용
@Component
public class ProductDao { // ProductDao라는 클래스 선언
public List<Product> getAllProducts() throws Exception { // 클래스 선언
Class.forName("com.mysql.cj.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/ureca?serverTimezone=UTC";
String user = "ureca";
String pw = "ureca";
String sql = "select * from product";
try (
Connection con = DriverManager.getConnection(url, user, pw); // 데베 연결
PreparedStatement stmt = con.prepareStatement(sql); // sql쿼리를 실행할 객체 생성
ResultSet rs = stmt.executeQuery() // sql 쿼리 실행하고 결과 집합 및 반환
) {
List<Product> list = new ArrayList<>(); // Product 객체들을 저장할 리스트 생성
while (rs.next()) {
int prodcode = rs.getInt("prodcode");
String prodname = rs.getString("prodname");
String pimg = rs.getString("pimg");
int price = rs.getInt("price");
list.add(new Product(prodcode, price, prodname, pimg));
}
return list;
}
}
}
Front 화면 구성
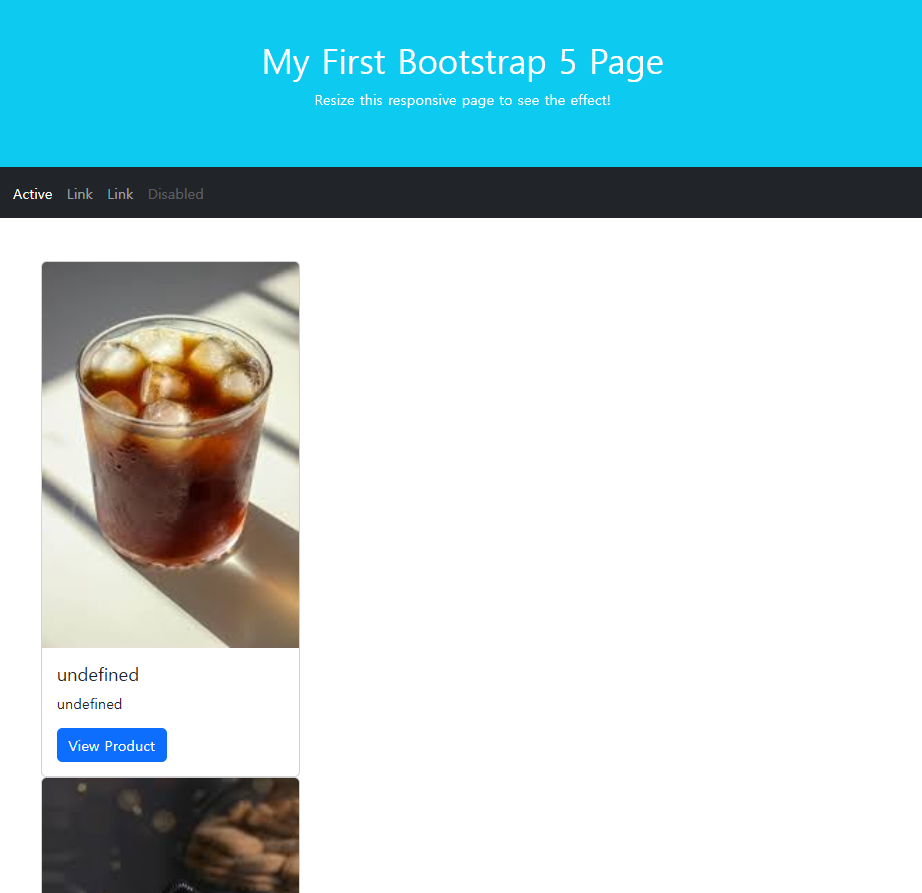
<!DOCTYPE html>
<html lang="en">
<head>
<title>Bootstrap 5 Website Example</title>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<link
href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css"
rel="stylesheet"
/>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/js/bootstrap.bundle.min.js"></script>
<style>
.fakeimg {
height: 200px;
background: #aaa;
}
</style>
</head>
<body>
<div class="p-5 bg-info text-white text-center">
<h1>My First Bootstrap 5 Page</h1>
<p>Resize this responsive page to see the effect!</p>
</div>
<nav class="navbar navbar-expand-sm bg-dark navbar-dark">
<div class="container-fluid">
<ul class="navbar-nav">
<li class="nav-item">
<a class="nav-link active" href="#">Active</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Link</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Link</a>
</li>
<li class="nav-item">
<a class="nav-link disabled" href="#">Disabled</a>
</li>
</ul>
</div>
</nav>
<div class="container mt-5">
<div class="row">
<div id="getAllProductsDiv"></div>
</div>
</div>
<div class="mt-5 p-4 bg-dark text-white text-center">
<p>Footer</p>
</div>
<script src="./js/index.js"></script>
</body>
</html>
window.onload = async () => {
let responseObj = await fetch('http://localhost:8080/getAllProducts', {
method: 'GET',
});
let arr = await responseObj.json();
let getAllProductsDiv = '';
arr.forEach((e) => {
getAllProductsDiv += `
<div class="card" style="width: 18rem;">
<img src="img/${e.pimg}" class="card-img-top" alt="...">
<div class="card-body">
<h5 class="card-title">${e.name}</h5>
<p class="card-text">${e.description}</p>
<a href="#" class="btn btn-primary">View Product</a>
</div>
</div>`;
});
document.getElementById('getAllProductsDiv').innerHTML = getAllProductsDiv;
};
더보기
이것이 풀스택의 세계인것인가..
오늘은 따라치기 바빴다..
728x90
'💡 URECA > 🗒️ 스터디 노트' 카테고리의 다른 글
[URECA] Day28 | Backend(3) (0) | 2025.03.06 |
---|---|
[URECA] Day 27 | Backend(2) (1) | 2025.03.05 |
[URECA] Day25 | SQL(1), JDBC (0) | 2025.02.28 |
[URECA] Day 24 | Git(2) (3) | 2025.02.27 |
[URECA] Day 23 | Git (0) | 2025.02.26 |